DEV/SpringBoot
SpringBoot Hello Controller
KO_O
2025. 3. 11. 13:56
Spring Boot는 Java 기반 애플리케이션을 쉽게 개발하고 배포할 수 있도록 도와주는 오픈소스 프레임워크예요.
최소한의 설정으로 실행 가능한 독립적인 애플리케이션을 빠르게 만들 수 있도록 도와줍니다 🙌
▶ Start?
모든 시작은... Hello를 출력하면서 시작하죠 ㅎㅎ
이번 글은 제가 처음 만들어본 Spring Boot Hello World 예제를 공유하려고 합니다.
프로젝트 구조를 이해하고, 간단한 컨트롤러를 직접 구현해본 경험을 정리해봤습니다 😊
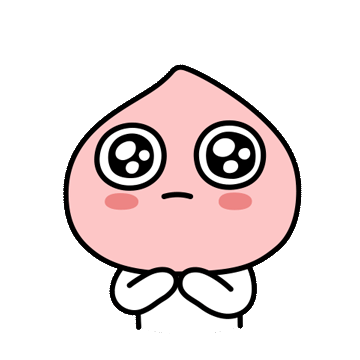
📌 GitHub Repository
👉 https://github.com/jo0ozip/SpringBoot-practice
📁 프로젝트 구조
SpringBoot-practice/
├── mvnw
├── pom.xml
├── src/
│ ├── main/
│ │ ├── java/com/example/springbootpractice/
│ │ │ ├── HelloController.java
│ │ │ └── SpringBootPracticeApplication.java
│ └── test/java/com/example/springbootpractice/
│ └── HelloControllerTest.java
💡 주요 코드 살펴보기
HelloController.java - /hello
요청을 처리하는 REST 컨트롤러입니다.
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "HELLO KIOR BLOG!";
}
}
- @RestController: REST 방식으로 응답을 반환하는 컨트롤러입니다.
- @GetMapping("/hello"):
/hello
로 들어오는 GET 요청을 처리합니다. - return "HELLO KIOR BLOG!": 텍스트 응답으로 해당 문구가 출력됩니다.
SpringBootPracticeApplication.java - 프로젝트의 시작점입니다.
@SpringBootApplication
public class SpringBootPracticeApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootPracticeApplication.class, args);
}
}
- @SpringBootApplication: 설정, 컴포넌트 스캔, 자동설정을 모두 포함하는 핵심 어노테이션입니다.
- main(): Spring Boot 애플리케이션의 시작 지점입니다.
🧪 테스트 코드: HelloControllerTest
Web Layer를 테스트하기 위한 단위 테스트 클래스입니다. 컨트롤러가 정상 동작하는지를 확인합니다.
@RunWith(SpringRunner.class)
@WebMvcTest(controllers = HelloController.class)
public class HelloControllerTest {
@Autowired
private MockMvc mvc;
@Test
public void hello가_리턴된다() throws Exception {
String hello = "HELLO KIOR BLOG!";
mvc.perform(get("/hello"))
.andExpect(status().isOk())
.andExpect(content().string(hello));
}
}
- @WebMvcTest: Controller만 로드해서 테스트하는 어노테이션입니다.
- MockMvc: 실제 서버 없이 API 요청/응답을 흉내내는 테스트 도구입니다.
- perform + andExpect:
/hello
요청을 보내고, 응답 상태와 내용이 예상과 일치하는지 검증합니다.
📌 이 테스트를 통해 실제 웹 서버를 띄우지 않고도 컨트롤러의 기능을 빠르게 확인할 수 있어요!
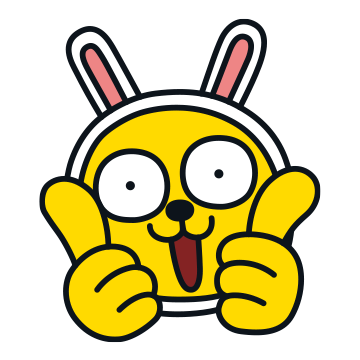
🚀 실행 방법
- Intellij > HelloControllerTest 실행
> Task :compileJava UP-TO-DATE > Task :processResources NO-SOURCE > Task :classes UP-TO-DATE > Task :compileTestJava UP-TO-DATE > Task :processTestResources NO-SOURCE > Task :testClasses UP-TO-DATE 13:44:15.149 [Test worker] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating CacheAwareContextLoaderDelegate from class [org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate] 13:44:15.152 [Test worker] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating BootstrapContext using constructor [public org.springframework.test.context.support.DefaultBootstrapContext(java.lang.Class,org.springframework.test.context.CacheAwareContextLoaderDelegate)] 13:44:15.165 [Test worker] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating TestContextBootstrapper for test class [com.kior.blog.springboot.web.HelloControllerTest] from class [org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper] 13:44:15.168 [Test worker] INFO org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - Neither @ContextConfiguration nor @ContextHierarchy found for test class [com.kior.blog.springboot.web.HelloControllerTest], using SpringBootContextLoader 13:44:15.170 [Test worker] DEBUG org.springframework.test.context.support.AbstractContextLoader - Did not detect default resource location for test class [com.kior.blog.springboot.web.HelloControllerTest]: class path resource [com/kior/blog/springboot/web/HelloControllerTest-context.xml] does not exist 13:44:15.170 [Test worker] DEBUG org.springframework.test.context.support.AbstractContextLoader - Did not detect default resource location for test class [com.kior.blog.springboot.web.HelloControllerTest]: class path resource [com/kior/blog/springboot/web/HelloControllerTestContext.groovy] does not exist 13:44:15.170 [Test worker] INFO org.springframework.test.context.support.AbstractContextLoader - Could not detect default resource locations for test class [com.kior.blog.springboot.web.HelloControllerTest]: no resource found for suffixes {-context.xml, Context.groovy}. 13:44:15.170 [Test worker] INFO org.springframework.test.context.support.AnnotationConfigContextLoaderUtils - Could not detect default configuration classes for test class [com.kior.blog.springboot.web.HelloControllerTest]: HelloControllerTest does not declare any static, non-private, non-final, nested classes annotated with @Configuration. 13:44:15.193 [Test worker] DEBUG org.springframework.test.context.support.ActiveProfilesUtils - Could not find an 'annotation declaring class' for annotation type [org.springframework.test.context.ActiveProfiles] and class [com.kior.blog.springboot.web.HelloControllerTest] 13:44:15.217 [Test worker] DEBUG org.springframework.context.annotation.ClassPathScanningCandidateComponentProvider - Identified candidate component class: file [/Users/kior/IdeaProjects/my-springboot/build/classes/java/main/com/kior/blog/springboot/Application.class] 13:44:15.218 [Test worker] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper - Found @SpringBootConfiguration com.kior.blog.springboot.Application for test class com.kior.blog.springboot.web.HelloControllerTest 13:44:15.219 [Test worker] DEBUG org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - @TestExecutionListeners is not present for class [com.kior.blog.springboot.web.HelloControllerTest]: using defaults. 13:44:15.219 [Test worker] INFO org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - Loaded default TestExecutionListener class names from location [META-INF/spring.factories]: [org.springframework.boot.test.autoconfigure.restdocs.RestDocsTestExecutionListener, org.springframework.boot.test.autoconfigure.web.client.MockRestServiceServerResetTestExecutionListener, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcPrintOnlyOnFailureTestExecutionListener, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverTestExecutionListener, org.springframework.boot.test.autoconfigure.webservices.client.MockWebServiceServerTestExecutionListener, org.springframework.boot.test.mock.mockito.MockitoTestExecutionListener, org.springframework.boot.test.mock.mockito.ResetMocksTestExecutionListener, org.springframework.test.context.web.ServletTestExecutionListener, org.springframework.test.context.support.DirtiesContextBeforeModesTestExecutionListener, org.springframework.test.context.event.ApplicationEventsTestExecutionListener, org.springframework.test.context.support.DependencyInjectionTestExecutionListener, org.springframework.test.context.support.DirtiesContextTestExecutionListener, org.springframework.test.context.transaction.TransactionalTestExecutionListener, org.springframework.test.context.jdbc.SqlScriptsTestExecutionListener, org.springframework.test.context.event.EventPublishingTestExecutionListener] 13:44:15.223 [Test worker] DEBUG org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - Skipping candidate TestExecutionListener [org.springframework.test.context.transaction.TransactionalTestExecutionListener] due to a missing dependency. Specify custom listener classes or make the default listener classes and their required dependencies available. Offending class: [org/springframework/transaction/interceptor/TransactionAttributeSource] 13:44:15.223 [Test worker] DEBUG org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - Skipping candidate TestExecutionListener [org.springframework.test.context.jdbc.SqlScriptsTestExecutionListener] due to a missing dependency. Specify custom listener classes or make the default listener classes and their required dependencies available. Offending class: [org/springframework/transaction/interceptor/TransactionAttribute] 13:44:15.223 [Test worker] INFO org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper - Using TestExecutionListeners: [org.springframework.test.context.web.ServletTestExecutionListener@32cb636e, org.springframework.test.context.support.DirtiesContextBeforeModesTestExecutionListener@63cd604c, org.springframework.test.context.event.ApplicationEventsTestExecutionListener@40dd3977, org.springframework.boot.test.mock.mockito.MockitoTestExecutionListener@3a4e343, org.springframework.boot.test.autoconfigure.SpringBootDependencyInjectionTestExecutionListener@6a1d204a, org.springframework.test.context.support.DirtiesContextTestExecutionListener@62dae245, org.springframework.test.context.event.EventPublishingTestExecutionListener@4b6579e8, org.springframework.boot.test.autoconfigure.restdocs.RestDocsTestExecutionListener@6fff253c, org.springframework.boot.test.autoconfigure.web.client.MockRestServiceServerResetTestExecutionListener@6c6357f9, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcPrintOnlyOnFailureTestExecutionListener@591e58fa, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverTestExecutionListener@3954d008, org.springframework.boot.test.autoconfigure.webservices.client.MockWebServiceServerTestExecutionListener@2f94c4db, org.springframework.boot.test.mock.mockito.ResetMocksTestExecutionListener@593e824f] 13:44:15.225 [Test worker] DEBUG org.springframework.test.context.support.AbstractDirtiesContextTestExecutionListener - Before test class: context [DefaultTestContext@7876d598 testClass = HelloControllerTest, testInstance = [null], testMethod = [null], testException = [null], mergedContextConfiguration = [WebMergedContextConfiguration@4a3e3e8b testClass = HelloControllerTest, locations = '{}', classes = '{class com.kior.blog.springboot.Application}', contextInitializerClasses = '[]', activeProfiles = '{}', propertySourceLocations = '{}', propertySourceProperties = '{org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper=true}', contextCustomizers = set[org.springframework.boot.test.autoconfigure.OverrideAutoConfigurationContextCustomizerFactory$DisableAutoConfigurationContextCustomizer@b6b1987, org.springframework.boot.test.autoconfigure.actuate.metrics.MetricsExportContextCustomizerFactory$DisableMetricExportContextCustomizer@7c541c15, org.springframework.boot.test.autoconfigure.filter.TypeExcludeFiltersContextCustomizer@8c8e9f86, org.springframework.boot.test.autoconfigure.properties.PropertyMappingContextCustomizer@2b1e51d4, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverContextCustomizerFactory$Customizer@67ab1c47, [ImportsContextCustomizer@5af28b27 key = [org.springframework.boot.autoconfigure.task.TaskExecutionAutoConfiguration, org.springframework.boot.autoconfigure.cache.CacheAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.HttpEncodingAutoConfiguration, org.springframework.boot.autoconfigure.validation.ValidationAutoConfiguration, org.springframework.boot.autoconfigure.context.MessageSourceAutoConfiguration, org.springframework.boot.autoconfigure.hateoas.HypermediaAutoConfiguration, org.springframework.boot.autoconfigure.data.web.SpringDataWebAutoConfiguration, org.springframework.boot.autoconfigure.jackson.JacksonAutoConfiguration, org.springframework.boot.test.autoconfigure.web.reactive.WebTestClientAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.SecurityAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.SecurityFilterAutoConfiguration, org.springframework.boot.autoconfigure.http.HttpMessageConvertersAutoConfiguration, org.springframework.boot.autoconfigure.security.oauth2.client.servlet.OAuth2ClientAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcSecurityConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcWebClientAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcWebDriverAutoConfiguration, org.springframework.boot.autoconfigure.thymeleaf.ThymeleafAutoConfiguration, org.springframework.boot.autoconfigure.gson.GsonAutoConfiguration, org.springframework.boot.autoconfigure.jsonb.JsonbAutoConfiguration, org.springframework.boot.autoconfigure.freemarker.FreeMarkerAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.WebMvcAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration, org.springframework.boot.autoconfigure.security.oauth2.resource.servlet.OAuth2ResourceServerAutoConfiguration, org.springframework.boot.autoconfigure.groovy.template.GroovyTemplateAutoConfiguration, org.springframework.boot.autoconfigure.mustache.MustacheAutoConfiguration]], org.springframework.boot.test.context.filter.ExcludeFilterContextCustomizer@16ecee1, org.springframework.boot.test.json.DuplicateJsonObjectContextCustomizerFactory$DuplicateJsonObjectContextCustomizer@4a3329b9, org.springframework.boot.test.mock.mockito.MockitoContextCustomizer@0, org.springframework.boot.test.context.SpringBootTestArgs@1, org.springframework.boot.test.context.SpringBootTestWebEnvironment@0], resourceBasePath = 'src/main/webapp', contextLoader = 'org.springframework.boot.test.context.SpringBootContextLoader', parent = [null]], attributes = map[[empty]]], class annotated with @DirtiesContext [false] with mode [null]. 13:44:15.228 [Test worker] DEBUG org.springframework.test.context.support.DependencyInjectionTestExecutionListener - Performing dependency injection for test context [[DefaultTestContext@7876d598 testClass = HelloControllerTest, testInstance = com.kior.blog.springboot.web.HelloControllerTest@4a067c25, testMethod = [null], testException = [null], mergedContextConfiguration = [WebMergedContextConfiguration@4a3e3e8b testClass = HelloControllerTest, locations = '{}', classes = '{class com.kior.blog.springboot.Application}', contextInitializerClasses = '[]', activeProfiles = '{}', propertySourceLocations = '{}', propertySourceProperties = '{org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTestContextBootstrapper=true}', contextCustomizers = set[org.springframework.boot.test.autoconfigure.OverrideAutoConfigurationContextCustomizerFactory$DisableAutoConfigurationContextCustomizer@b6b1987, org.springframework.boot.test.autoconfigure.actuate.metrics.MetricsExportContextCustomizerFactory$DisableMetricExportContextCustomizer@7c541c15, org.springframework.boot.test.autoconfigure.filter.TypeExcludeFiltersContextCustomizer@8c8e9f86, org.springframework.boot.test.autoconfigure.properties.PropertyMappingContextCustomizer@2b1e51d4, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverContextCustomizerFactory$Customizer@67ab1c47, [ImportsContextCustomizer@5af28b27 key = [org.springframework.boot.autoconfigure.task.TaskExecutionAutoConfiguration, org.springframework.boot.autoconfigure.cache.CacheAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.HttpEncodingAutoConfiguration, org.springframework.boot.autoconfigure.validation.ValidationAutoConfiguration, org.springframework.boot.autoconfigure.context.MessageSourceAutoConfiguration, org.springframework.boot.autoconfigure.hateoas.HypermediaAutoConfiguration, org.springframework.boot.autoconfigure.data.web.SpringDataWebAutoConfiguration, org.springframework.boot.autoconfigure.jackson.JacksonAutoConfiguration, org.springframework.boot.test.autoconfigure.web.reactive.WebTestClientAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.SecurityAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.SecurityFilterAutoConfiguration, org.springframework.boot.autoconfigure.http.HttpMessageConvertersAutoConfiguration, org.springframework.boot.autoconfigure.security.oauth2.client.servlet.OAuth2ClientAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcSecurityConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcWebClientAutoConfiguration, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcWebDriverAutoConfiguration, org.springframework.boot.autoconfigure.thymeleaf.ThymeleafAutoConfiguration, org.springframework.boot.autoconfigure.gson.GsonAutoConfiguration, org.springframework.boot.autoconfigure.jsonb.JsonbAutoConfiguration, org.springframework.boot.autoconfigure.freemarker.FreeMarkerAutoConfiguration, org.springframework.boot.autoconfigure.web.servlet.WebMvcAutoConfiguration, org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration, org.springframework.boot.autoconfigure.security.oauth2.resource.servlet.OAuth2ResourceServerAutoConfiguration, org.springframework.boot.autoconfigure.groovy.template.GroovyTemplateAutoConfiguration, org.springframework.boot.autoconfigure.mustache.MustacheAutoConfiguration]], org.springframework.boot.test.context.filter.ExcludeFilterContextCustomizer@16ecee1, org.springframework.boot.test.json.DuplicateJsonObjectContextCustomizerFactory$DuplicateJsonObjectContextCustomizer@4a3329b9, org.springframework.boot.test.mock.mockito.MockitoContextCustomizer@0, org.springframework.boot.test.context.SpringBootTestArgs@1, org.springframework.boot.test.context.SpringBootTestWebEnvironment@0], resourceBasePath = 'src/main/webapp', contextLoader = 'org.springframework.boot.test.context.SpringBootContextLoader', parent = [null]], attributes = map['org.springframework.test.context.event.ApplicationEventsTestExecutionListener.recordApplicationEvents' -> false]]]. . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v2.7.18) 2025-03-11 13:44:15.355 INFO 32804 --- [ Test worker] c.k.b.s.web.HelloControllerTest : Starting HelloControllerTest using Java 1.8.0_431 on MACKIOR.local with PID 32804 (started by kior in /Users/kior/IdeaProjects/my-springboot) 2025-03-11 13:44:15.356 INFO 32804 --- [ Test worker] c.k.b.s.web.HelloControllerTest : No active profile set, falling back to 1 default profile: "default" 2025-03-11 13:44:15.715 INFO 32804 --- [ Test worker] o.s.b.t.m.w.SpringBootMockServletContext : Initializing Spring TestDispatcherServlet '' 2025-03-11 13:44:15.715 INFO 32804 --- [ Test worker] o.s.t.web.servlet.TestDispatcherServlet : Initializing Servlet '' 2025-03-11 13:44:15.716 INFO 32804 --- [ Test worker] o.s.t.web.servlet.TestDispatcherServlet : Completed initialization in 1 ms 2025-03-11 13:44:15.729 INFO 32804 --- [ Test worker] c.k.b.s.web.HelloControllerTest : Started HelloControllerTest in 0.489 seconds (JVM running for 0.849) > Task :test Deprecated Gradle features were used in this build, making it incompatible with Gradle 9.0. You can use '--warning-mode all' to show the individual deprecation warnings and determine if they come from your own scripts or plugins. For more on this, please refer to https://docs.gradle.org/8.10/userguide/command_line_interface.html#sec:command_line_warnings in the Gradle documentation. BUILD SUCCESSFUL in 4s 3 actionable tasks: 1 executed, 2 up-to-date 오후 1:44:15: 실행이 완료되었습니다 ':test --tests "com.kior.blog.springboot.web.HelloControllerTest"'.
- intellij > Application 실행
/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/bin/java -XX:TieredStopAtLevel=1 -noverify -Dspring.output.ansi.enabled=always -Dcom.sun.management.jmxremote -Dspring.jmx.enabled=true -Dspring.liveBeansView.mbeanDomain -Dspring.application.admin.enabled=true -Dmanagement.endpoints.jmx.exposure.include=* -javaagent:/Applications/IntelliJ IDEA.app/Contents/lib/idea_rt.jar=57265:/Applications/IntelliJ IDEA.app/Contents/bin -Dfile.encoding=UTF-8 -classpath /Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/charsets.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/deploy.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/cldrdata.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/dnsns.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/jaccess.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/jfxrt.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/localedata.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/nashorn.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/sunec.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/sunjce_provider.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/sunpkcs11.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/ext/zipfs.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/javaws.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/jce.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/jfr.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/jfxswt.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/jsse.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/management-agent.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/plugin.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/resources.jar:/Users/kior/iTgym/Intellij/jdk1.8.0_431.jdk/Contents/Home/jre/lib/rt.jar:/Users/kior/IdeaProjects/my-springboot/build/classes/java/main:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-starter-web/2.7.18/dd62ea85098187b4604e78dc15a7ff87dba173d/spring-boot-starter-web-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-starter-json/2.7.18/b6d9ed5cae0c1929a9e561bf4799a3dc93a10db1/spring-boot-starter-json-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-starter/2.7.18/e56b75105f9ace6df154fd47eeeeadc2f5791e56/spring-boot-starter-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-starter-tomcat/2.7.18/c56e50e006448e75a8bde595dbc754ba294389af/spring-boot-starter-tomcat-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-webmvc/5.3.31/45754d056effe8257a012f6b98ed5454cf1e8960/spring-webmvc-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-web/5.3.31/3bf73c385a1f2f4a0d482149d6a205e854cec497/spring-web-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.datatype/jackson-datatype-jsr310/2.13.5/8ba3b868e81d7fc6ead686bd2353859b111d9eaf/jackson-datatype-jsr310-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.module/jackson-module-parameter-names/2.13.5/a401a99e7a45450fd3ef76e82ba39005fd1a8c22/jackson-module-parameter-names-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.datatype/jackson-datatype-jdk8/2.13.5/1278f38160812811c56eb77f67213662ed1c7a2e/jackson-datatype-jdk8-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.core/jackson-databind/2.13.5/aa95e46dbc32454f3983221d420e78ef19ddf844/jackson-databind-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-autoconfigure/2.7.18/9cf147c6ca274c75b32556acdcba5a1de081ebcd/spring-boot-autoconfigure-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot/2.7.18/f6dbdd8da7c2bded63dff9b1f48d01a4923f20a0/spring-boot-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework.boot/spring-boot-starter-logging/2.7.18/19f7c255ba5255116f58c3bbaf52c7b88ea6af3e/spring-boot-starter-logging-2.7.18.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/jakarta.annotation/jakarta.annotation-api/1.3.5/59eb84ee0d616332ff44aba065f3888cf002cd2d/jakarta.annotation-api-1.3.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-core/5.3.31/368e76f732a3c331b970f69cafec1525d27b34d3/spring-core-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.yaml/snakeyaml/1.30/8fde7fe2586328ac3c68db92045e1c8759125000/snakeyaml-1.30.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.apache.tomcat.embed/tomcat-embed-websocket/9.0.83/9af4b7450296bb4eff93b2ee3e52ab69d07512e4/tomcat-embed-websocket-9.0.83.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.apache.tomcat.embed/tomcat-embed-core/9.0.83/d771e4343b0515c67dab2a09fe02f5d47550153f/tomcat-embed-core-9.0.83.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.apache.tomcat.embed/tomcat-embed-el/9.0.83/b0cdada70099c25f45fceb48e1ebce60d138a5ce/tomcat-embed-el-9.0.83.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-context/5.3.31/a2d6e76507f037ad835e8c2288dfedf28981999f/spring-context-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-aop/5.3.31/3be929dbdb5f4516919ad09a3d3720d779bb65d9/spring-aop-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-beans/5.3.31/d27258849071b3b268ecc388eca35bbfcc586448/spring-beans-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-expression/5.3.31/55637af1b186d1008890980c2876c5fc83599756/spring-expression-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.core/jackson-annotations/2.13.5/136f77ab424f302c9e27230b4482e8000e142edf/jackson-annotations-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/com.fasterxml.jackson.core/jackson-core/2.13.5/d07c97d3de9ea658caf1ff1809fd9de930a286a/jackson-core-2.13.5.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/ch.qos.logback/logback-classic/1.2.12/d4dee19148dccb177a0736eb2027bd195341da78/logback-classic-1.2.12.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-to-slf4j/2.17.2/17dd0fae2747d9a28c67bc9534108823d2376b46/log4j-to-slf4j-2.17.2.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.slf4j/jul-to-slf4j/1.7.36/ed46d81cef9c412a88caef405b58f93a678ff2ca/jul-to-slf4j-1.7.36.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.springframework/spring-jcl/5.3.31/e7ab9ee590a195415dd6b898440d776b4c8db78c/spring-jcl-5.3.31.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/ch.qos.logback/logback-core/1.2.12/1d8e51a698b138065d73baefb4f94531faa323cb/logback-core-1.2.12.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.slf4j/slf4j-api/1.7.36/6c62681a2f655b49963a5983b8b0950a6120ae14/slf4j-api-1.7.36.jar:/Users/kior/.gradle/caches/modules-2/files-2.1/org.apache.logging.log4j/log4j-api/2.17.2/f42d6afa111b4dec5d2aea0fe2197240749a4ea6/log4j-api-2.17.2.jar com.kior.blog.springboot.Application . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v2.7.18) 2025-03-11 13:54:07.152 INFO 32854 --- [ main] com.kior.blog.springboot.Application : Starting Application using Java 1.8.0_431 on MACKIOR.local with PID 32854 (/Users/kior/IdeaProjects/my-springboot/build/classes/java/main started by kior in /Users/kior/IdeaProjects/my-springboot) 2025-03-11 13:54:07.153 INFO 32854 --- [ main] com.kior.blog.springboot.Application : No active profile set, falling back to 1 default profile: "default" 2025-03-11 13:54:07.372 INFO 32854 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http) 2025-03-11 13:54:07.374 INFO 32854 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat] 2025-03-11 13:54:07.374 INFO 32854 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.83] 2025-03-11 13:54:07.414 INFO 32854 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext 2025-03-11 13:54:07.414 INFO 32854 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 249 ms 2025-03-11 13:54:07.502 INFO 32854 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path '' 2025-03-11 13:54:07.505 INFO 32854 --- [ main] com.kior.blog.springboot.Application : Started Application in 0.447 seconds (JVM running for 0.618)
- 브라우저에서 접속
http://localhost:8080/hello
✅ 결과: HELLO KIOR BLOG!
문장이 출력됩니다 🎉
📝 마무리
누구나 Hello부터 시작하듯, 이 프로젝트는 Spring Boot 입문의 첫걸음이었습니다 :)
매번 개발서의 앞만 건드렸는데... 작지만 의미 있는 한 발자국! 꾸준하게 추가하며 발전시켜보겠습니다 😄
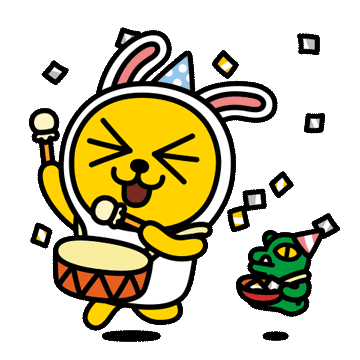